functions in C
The function in C language is also known as procedure or subroutine in other programming languages.To perform any task, we can create function. A function can be called many times. It provides modularity and code reusability.
Advantage of functions in C
There are many advantages of functions.1) Code Reusability
By creating functions in C, you can call it many times. So we don't need to write the same code again and again.2) Code optimization
It makes the code optimized, we don't need to write much code.Suppose, you have to check 3 numbers (781, 883 and 531) whether it is prime number or not. Without using function, you need to write the prime number logic 3 times. So, there is repetition of code.
But if you use functions, you need to write the logic only once and you can reuse it several times.
Types of Functions
There are two types of functions in C programming:- Library Functions: are the functions which are declared in the C header files such as scanf(), printf(), gets(), puts(), ceil(), floor() etc.
- User-defined functions: are the functions which are created by the C programmer, so that he/she can use it many times. It reduces complexity of a big program and optimizes the code.
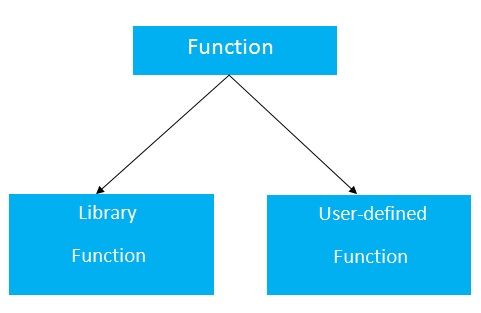
How user-defined function works?
#include <stdio.h> void functionName() { ... .. ... ... .. ... } int main() { ... .. ... ... .. ... functionName(); ... .. ... ... .. ... }
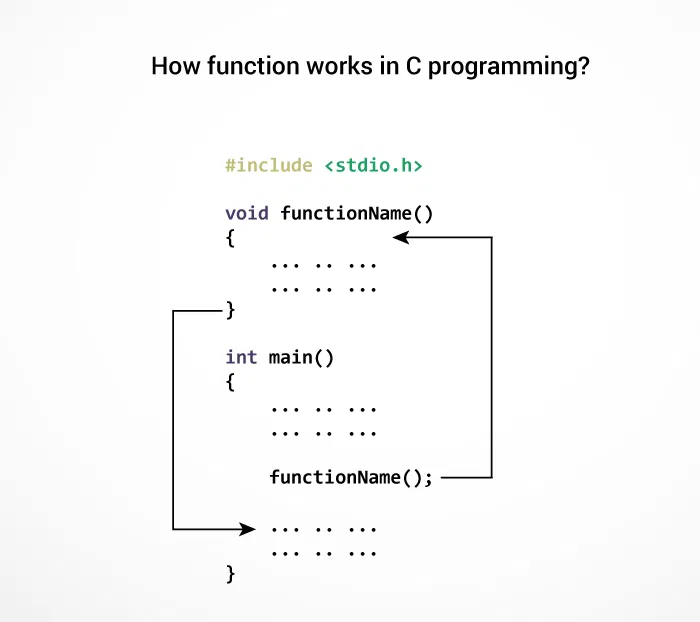
1-Function Prototype(Function declaration)
SyntexdataType functionName (Parameter List); Example
int addition();
2-Function definition
SyntexreturnType functionName(Function arguments) { //body of the function } Example int addition() { }
3-Function Call
#include<stdio.h>/* function declaration */
int addition();
int main()
{
/* local variable definition */
int answer;
/* calling a function to get addition value */
answer = addition();
printf("The addition of two numbers is: %d\n",answer);
return 0;
}
/* function returning the addition of two numbers */
int addition()
{
/* local variable definition */
int num1 = 10, num2 = 5;
return num1+num2;
}
Return Value
A C function may or may not return a value from the function. If you don't have to return any value from the function, use void for the return type.Let's see a simple example of C function that doesn't return any value from the function.
Example without return value:
- void hello(){
- printf("hello c");
- }
Let's see a simple example of C function that returns int value from the function.
Example with return value:
- int get(){
- return 10;
- }
- float get(){
- return 10.2;
- }
Parameters in C Function
A c function may have 0 or more parameters. You can have any type of parameter in C program such as int, float, char etc. The parameters are also known as formal arguments.Example of a function that has 0 parameter:
- void hello(){
- printf("hello c");
- }
- int cube(int n){
- return n*n*n;
- }
- int add(int a, int b){
- return a+b;
- }
Types of Function calls in C
Functions are called by their names. If the function is without argument, it can be called directly using its name. But for functions with arguments, we have two ways to call them,- Call by Value
- Call by Reference
Call by Value
In this calling technique we pass the values of arguments which are stored or copied into the formal parameters of functions. Hence, the original values are unchanged only the parameters inside function changes.void calc(int x); int main() { int x = 10; calc(x); printf("%d", x); } void calc(int x) { x = x + 10 ; }Output : 10
In this case the actual variable x is not changed, because we pass argument by value, hence a copy of x is passed, which is changed, and that copied value is destroyed as the function ends(goes out of scope). So the variable x inside main() still has a value 10.
But we can change this program to modify the original x, by making the function calc() return a value, and storing that value in x.
int calc(int x); int main() { int x = 10; x = calc(x); printf("%d", x); } int calc(int x) { x = x + 10 ; return x; }Output : 20
Call by Reference
In this we pass the address of the variable as arguments. In this case the formal parameter can be taken as a reference or a pointer, in both the case they will change the values of the original variable.void calc(int *p); int main() { int x = 10; calc(&x); // passing address of x as argument printf("%d", x); } void calc(int *p) { *p = *p + 10; }Output : 20
NOTE : If you do not have a prior knowledge of pointers, do study Pointers first.
Passing Array to Function
Whenever we need to pass a list of elements as argument to the function, it is prefered to do so using an array. But how can we pass an array as argument ? Lets see how to do it.Declaring Function with array in parameter list
There are two possible ways to do so, one will lead to call by value and the other is used to perform call be reference.- We can either have an array in parameter.
int sum (int arr[]);
- Or, we can have a pointer in the parameter list, to hold base address of array.
int sum (int* ptr);
We will study this in detail later when we will study pointers.
Returning Array from function
We dont't return an array from functions, rather we return a pointer holding the base address of the array to be returned. But we must, make sure that the array exists after the function ends.int* sum (int x[]) { //statements return x ; }We will discuss about this when we will study pointers with arrays.
Example : Create a function to sort an Array of elements
void sorting(int x[],int y) { int i, j, temp ; for(i=1; i<=y-1; i++) { for(j=0; j< y-i; j++) { if(x[j] > x[j+1]) { temp = x[j]; x[j] = x[j+1]; x[j+1] = temp; } } } for(i=0;i<5;i++) { printf("\t%d",x[i]); } }In the above example,
- return type is void, that means function does not return any thing.
- Sorting is the function name.
- int x[ ] and int y is the parameter list.
- int i, j, temp inside curly braces are the local variable declaraction.
Recursion in C
When function is called within the same function, it is known as recursion in C. The function which calls the same function, is known as recursive function.A function that calls itself, and doesn't perform any task after function call, is know as tail recursion. In tail recursion, we generally call the same function with return statement. An example of tail recursion is given below.
Let's see a simple example of recursion.
recursionfunction(){
recursionfunction();//calling self function
}
Example of tail recursion in C
Let's see an example to print factorial number using tail recursion in C language.#include<stdio.h>
#include<conio.h>
int factorial (int n)
{
if ( n < 0)
return -1; /*Wrong value*/
if (n == 0)
return 1; /*Terminating condition*/
return (n * factorial (n -1));
}
void main(){
int fact=0;
clrscr();
fact=factorial(5);
printf("\n factorial of 5 is %d",fact);
getch();
}
Output
factorial of 5 is 120

Types of User-defined Functions in C Programming
The 4 programs below check whether an integer entered by the user is a prime number or not. And, all these programs generate the same output.Example #1: No arguments passed and no return Value
#include <stdio.h> void checkPrimeNumber(); int main() { checkPrimeNumber(); // no argument is passed to prime() return 0; } // return type of the function is void becuase no value is returned from the function void checkPrimeNumber() { int n, i, flag=0; printf("Enter a positive integer: "); scanf("%d",&n); for(i=2; i <= n/2; ++i) { if(n%i == 0) { flag = 1; } } if (flag == 1) printf("%d is not a prime number.", n); else printf("%d is a prime number.", n); }
Example #2: No arguments passed but a return value
#include <stdio.h> int getInteger(); int main() { int n, i, flag = 0; // no argument is passed to the function // the value returned from the function is assigned to n n = getInteger(); for(i=2; i<=n/2; ++i) { if(n%i==0) { flag = 1; break; } } if (flag == 1) printf("%d is not a prime number.", n); else printf("%d is a prime number.", n); return 0; } // getInteger() function returns integer entered by the user int getInteger() { int n; printf("Enter a positive integer: "); scanf("%d",&n); return n; }
Example #3: Argument passed but no return value
#include <stdio.h> void checkPrimeAndDisplay(int n); int main() { int n; printf("Enter a positive integer: "); scanf("%d",&n); // n is passed to the function checkPrimeAndDisplay(n); return 0; } // void indicates that no value is returned from the function void checkPrimeAndDisplay(int n) { int i, flag = 0; for(i=2; i <= n/2; ++i) { if(n%i == 0) { flag = 1; break; } } if(flag == 1) printf("%d is not a prime number.",n); else printf("%d is a prime number.", n); }
Example #4: Argument passed and a return value
#include <stdio.h> int checkPrimeNumber(int n); int main() { int n, flag; printf("Enter a positive integer: "); scanf("%d",&n); // n is passed to the checkPrimeNumber() function // the value returned from the function is assigned to flag variable flag = checkPrimeNumber(n); if(flag==1) printf("%d is not a prime number",n); else printf("%d is a prime number",n); return 0; } // integer is returned from the function int checkPrimeNumber(int n) { /* Integer value is returned from function checkPrimeNumber() */ int i; for(i=2; i <= n/2; ++i) { if(n%i == 0) return 1; } return 0; }
No comments:
Post a Comment
Please write your view and suggestion....